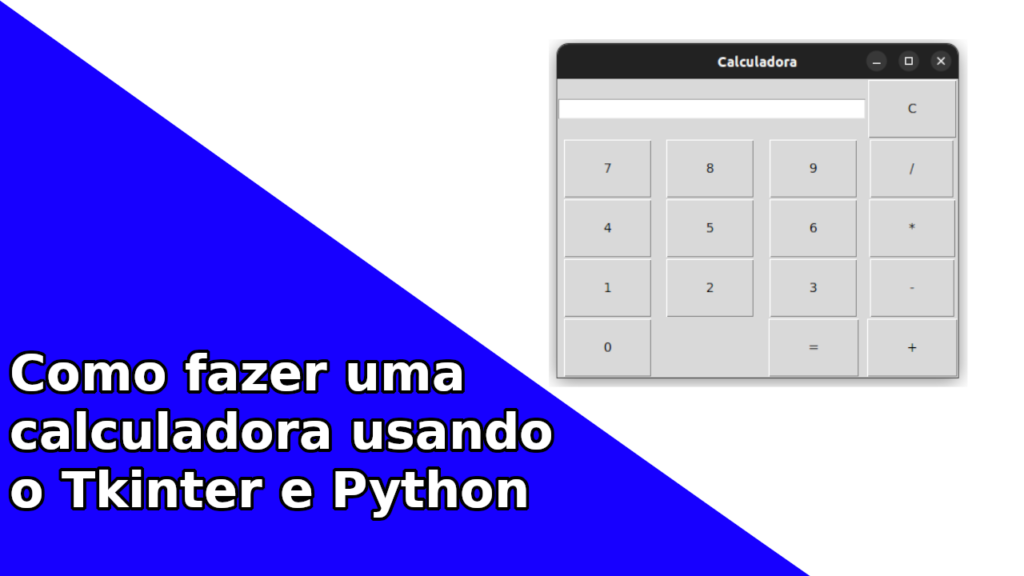
Neste post vamos ver como fazer uma calculadora usando Python e Tkinter. No vídeo a seguir é apresentado os detalhes do projeto:
Código
Abaixo é apresentado o código da calculadora que foi apresentado no vídeo:
from tkinter import *
root = Tk()
root.title ("Calculadora")
disp = Entry (root, width = 40)
disp.grid (row = 0, column = 0, columnspan = 3)
def button_number(num):
atual = disp.get()
disp.delete(0, END)
disp.insert(0, str(atual) + str(num))
def soma():
memoria = disp.get()
global mem
global operacao
operacao = 'soma'
mem = int (memoria)
disp.delete(0, END)
def subtrai():
memoria = disp.get()
global mem
global operacao
operacao = 'sub'
mem = int (memoria)
disp.delete(0, END)
def multiplica():
memoria = disp.get()
global mem
global operacao
operacao = 'mul'
mem = int (memoria)
disp.delete(0, END)
def divide():
memoria = disp.get()
global mem
global operacao
operacao = 'div'
mem = int (memoria)
disp.delete(0, END)
def igual():
numero = disp.get()
disp.delete(0, END)
if operacao == 'soma':
disp.insert(0, mem + int (numero))
if operacao == 'sub':
disp.insert(0, mem - int (numero))
if operacao == 'mul':
disp.insert(0, mem * int (numero))
if operacao == 'div':
disp.insert(0, mem / int (numero))
def limpar():
disp.delete(0, END)
# Teclado
bot_1 = Button(root, text = "1", padx = 40, pady = 20, command = lambda: button_number(1))
bot_2 = Button(root, text = "2", padx = 40, pady = 20, command = lambda: button_number(2))
bot_3 = Button(root, text = "3", padx = 40, pady = 20, command = lambda: button_number(3))
bot_4 = Button(root, text = "4", padx = 40, pady = 20, command = lambda: button_number(4))
bot_5 = Button(root, text = "5", padx = 40, pady = 20, command = lambda: button_number(5))
bot_6 = Button(root, text = "6", padx = 40, pady = 20, command = lambda: button_number(6))
bot_7 = Button(root, text = "7", padx = 40, pady = 20, command = lambda: button_number(7))
bot_8 = Button(root, text = "8", padx = 40, pady = 20, command = lambda: button_number(8))
bot_9 = Button(root, text = "9", padx = 40, pady = 20, command = lambda: button_number(9))
bot_0 = Button(root, text = "0", padx = 40, pady = 20, command = lambda: button_number(0))
bot_sum = Button(root, text = "+", padx = 40, pady = 20, command = soma)
bot_sub = Button(root, text = "-", padx = 40, pady = 20, command = subtrai)
bot_mul = Button(root, text = "*", padx = 40, pady = 20, command = multiplica)
bot_div = Button(root, text = "/", padx = 40, pady = 20, command = divide)
bot_equal = Button(root, text = "=", padx = 40, pady = 20, command = igual)
bot_clear = Button(root, text = "C", padx = 40, pady = 20, command = limpar)
#grids do teclado
bot_1.grid (row = 3, column = 0)
bot_2.grid (row = 3, column = 1)
bot_3.grid (row = 3, column = 2)
bot_4.grid (row = 2, column = 0)
bot_5.grid (row = 2, column = 1)
bot_6.grid (row = 2, column = 2)
bot_7.grid (row = 1, column = 0)
bot_8.grid (row = 1, column = 1)
bot_9.grid (row = 1, column = 2)
bot_0.grid (row = 4, column = 0)
bot_sum.grid (row = 4, column = 3)
bot_sub.grid (row = 3, column = 3)
bot_mul.grid (row = 2, column = 3)
bot_div.grid (row = 1, column = 3)
bot_equal.grid (row = 4, column = 2)
bot_clear.grid (row = 0, column = 3)
root.mainloop()
Neste post vimos como fazer uma calculadora usando Python, até o próximo post.